I recently started working on this again. Past 2 days. It now "works". Note that the word "works" is in scare quotes for a reason. The load code still needs complex type enumeration re-alignment and I've got to test every function and look for crashes.
I need some people who are willing to try to test this. There is a lot of work to do, and it's buggy as hell. It can be finished, but it needs lots of testing.
Work remaining:
- Test all functions
- Design scope/module system (current implementation is just workable)
- Redo hook system to handel return values better. Right now it has Call or CallAll where All preserves all returns and Call stops working after the first. This was good for a hook system laying ontop of a centralized script, but I've used this as part of the core, so it doesn't work outright.
- Fix Save/Load
Code: Select all
local _G = _G;
local hook = hook;
_G.PrintConsoleMessage("Loading GameMode.InstantAction");
module( "InstantAction" )
_G.MissionData.Text = "STRING";
hook.Add( "Update", "Chilli_Custom_Update", function( GameTurn )
if ( _G.MissionData.TargetTest == nil
or not _G.MissionData.TargetTest:IsAround() ) then
SpawnBox();
end
if GameTurn % 10 == 0 then
if _G.MissionData.TargetTestIsObjectified then
_G.MissionData.TargetTest:SetObjectiveOff();
else
_G.MissionData.TargetTest:SetObjectiveOn();
end
_G.MissionData.TargetTestIsObjectified = not _G.MissionData.TargetTestIsObjectified;
end
end )
function SpawnBox()
local player = _G.GetPlayerHandle(1);
local PlayerLocation = player:GetPositionV();
local vectorLoc = _G.SetCircularPos(PlayerLocation, _G.GetRandomFloat(10)+20, _G.GetRandomFloat(6.28318530718));
_G.MissionData.TargetTest = _G.BuildObject("ibcrat00", 2, vectorLoc);
_G.MissionData.TargetTestIsObjectified = false;
end
hook.Add( "InitialSetup", "Chilli_Custom_InitialSetup", function()
SpawnBox();
end )
_G.PrintConsoleMessage("Loadinged GameMode.InstantAction");
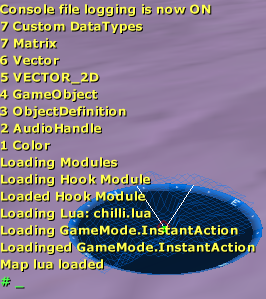
So, I need testers and people willing to help code this.
The entire code base is here: https://github.com/Nielk1/BZ2-LuaMission
The code _api.lua is over 5200 lines.
I can generate some documentation once I've got some people willing to help. I really need people who are willing to learn the LUA side or who know enough about the C side to help me resolve some of the bigger issues. I do all my coding for work in C# so my C is very rusty.